Analyze data with AI
You can use E2B Sandbox to run AI-generated code to analyze data. Here's how the AI data analysis workflow usually looks like:
- Your user has a dataset in CSV format or other formats.
- You prompt the LLM to generate code (usually Python) based on the user's data.
- The sandbox runs the AI-generated code and returns the results.
- You display the results to the user.
Example: Analyze CSV file with E2B and Claude 3.5 Sonnet
This short example will show you how to use E2B Sandbox to run AI-generated code to analyze CSV data.
Table of Contents
- Install dependencies
- Set your API keys
- Download example CSV file
- Initialize the sandbox and upload the dataset to the sandbox
- Prepare the method for running AI-generated code
- Prepare the prompt and initialize Anthropic client
- Connect the sandbox to the LLM with tool calling
- Parse the LLM response and run the AI-generated code in the sandbox
- Save the generated chart
- Run the code
- Full final code
1. Install dependencies
Install the E2B SDK and Claude SDK to your project by running the following command in your terminal.
npm i @e2b/code-interpreter @anthropic-ai/sdk dotenv
2. Set your API keys
- Get your E2B API key from E2B Dashboard.
- Get your Claude API key from Claude API Dashboard.
- Paste the keys into your
.env
file.
.env
E2B_API_KEY=e2b_***
ANTHROPIC_API_KEY=sk-ant-***
3. Download example CSV file
We'll be using the publicly available dataset of about 10,000 movies.
- Click the "Download" button at the top of the page.
- Select "Download as zip (2 MB)".
- Unzip the file and you should see
dataset.csv
file. Move it to the root of your project.
4. Initialize the sandbox and upload the dataset to the sandbox
We'll upload the dataset from the third step to the sandbox and save it as dataset.csv
file.
import 'dotenv/config'
import fs from 'fs'
import { Sandbox } from '@e2b/code-interpreter'
// Create sandbox
const sbx = await Sandbox.create()
// Upload the dataset to the sandbox
const content = fs.readFileSync('dataset.csv')
const datasetPathInSandbox = await sbx.files.write('dataset.csv', content)
5. Prepare the method for running AI-generated code
Add the following code to the file. Here we're adding the method for code execution.
// ... code from the previous step
async function runAIGeneratedCode(aiGeneratedCode: string) {
console.log('Running the code in the sandbox....')
const execution = await sbx.runCode(aiGeneratedCode)
console.log('Code execution finished!')
console.log(execution)
}
6. Prepare the prompt and initialize Anthropic client
The prompt we'll be using describes the dataset and the analysis we want to perform like this:
- Describe the columns in the CSV dataset.
- Ask the LLM what we want to analyze - here we want to analyze the vote average over time. We're asking for a chart as the output.
- Instruct the LLM to generate Python code for the data analysis.
import Anthropic from '@anthropic-ai/sdk'
const prompt = `
I have a CSV file about movies. It has about 10k rows. It's saved in the sandbox at ${dataset_path_in_sandbox.path}.
These are the columns:
- 'id': number, id of the movie
- 'original_language': string like "eng", "es", "ko", etc
- 'original_title': string that's name of the movie in the original language
- 'overview': string about the movie
- 'popularity': float, from 0 to 9137.939. It's not normalized at all and there are outliers
- 'release_date': date in the format yyyy-mm-dd
- 'title': string that's the name of the movie in english
- 'vote_average': float number between 0 and 10 that's representing viewers voting average
- 'vote_count': int for how many viewers voted
I want to better understand how the vote average has changed over the years. Write Python code that analyzes the dataset based on my request and produces right chart accordingly`
const anthropic = new Anthropic()
console.log('Waiting for the model response...')
const msg = await anthropic.messages.create({
model: 'claude-3-5-sonnet-20240620',
max_tokens: 1024,
messages: [{ role: 'user', content: prompt }],
})
7. Connect the sandbox to the LLM with tool calling
We'll use Claude's ability to use tools (function calling) to run the code in the sandbox.
The way we'll do it is by connecting the method for running AI-generated code we created in the previous step to the Claude model.
Update the initialization of the Anthropic client to include the tool use like this:
const msg = await anthropic.messages.create({
model: 'claude-3-5-sonnet-20240620',
max_tokens: 1024,
messages: [{ role: 'user', content: prompt }],
tools: [
{
name: 'run_python_code',
description: 'Run Python code',
input_schema: {
type: 'object',
properties: {
code: {
type: 'string',
description: 'The Python code to run',
},
},
required: ['code'],
},
},
],
})
8. Parse the LLM response and run the AI-generated code in the sandbox
Now we'll parse the msg
object to get the code from the LLM's response based on the tool we created in the previous step.
Once we have the code, we'll pass it to the runAIGeneratedCode
method in JavaScript or run_ai_generated_code
method in Python we created in the previous step to run the code in the sandbox.
// ... code from the previous steps
interface CodeRunToolInput {
code: string
}
for (const contentBlock of msg.content) {
if (contentBlock.type === 'tool_use') {
if (contentBlock.name === 'run_python_code') {
const code = (contentBlock.input as CodeRunToolInput).code
console.log('Will run following code in the sandbox', code)
// Execute the code in the sandbox
await runAIGeneratedCode(code)
}
}
}
9. Save the generated chart
When running code in the sandbox for data analysis, you can get different types of results. Including stdout, stderr, charts, tables, text, runtime errors, and more.
In this example we're specifically asking for a chart so we'll be looking for the chart in the results.
Let's update the runAIGeneratedCode
method in JavaScript and run_ai_generated_code
method in Python to check for the chart in the results and save it to the file.
async function runAIGeneratedCode(aiGeneratedCode: string) {
console.log('Running the code in the sandbox....')
const execution = await sbx.runCode(aiGeneratedCode)
console.log('Code execution finished!')
// First let's check if the code ran successfully.
if (execution.error) {
console.error('AI-generated code had an error.')
console.log(execution.error.name)
console.log(execution.error.value)
console.log(execution.error.traceback)
process.exit(1)
}
// Iterate over all the results and specifically check for png files that will represent the chart.
let resultIdx = 0
for (const result of execution.results) {
if (result.png) {
// Save the png to a file
// The png is in base64 format.
fs.writeFileSync(`chart-${resultIdx}.png`, result.png, { encoding: 'base64' })
console.log(`Chart saved to chart-${resultIdx}.png`)
resultIdx++
}
}
}
10. Run the code
Now you can run the whole code to see the results.
npx tsx index.ts
You should see the chart in the root of your project that will look similar to this:
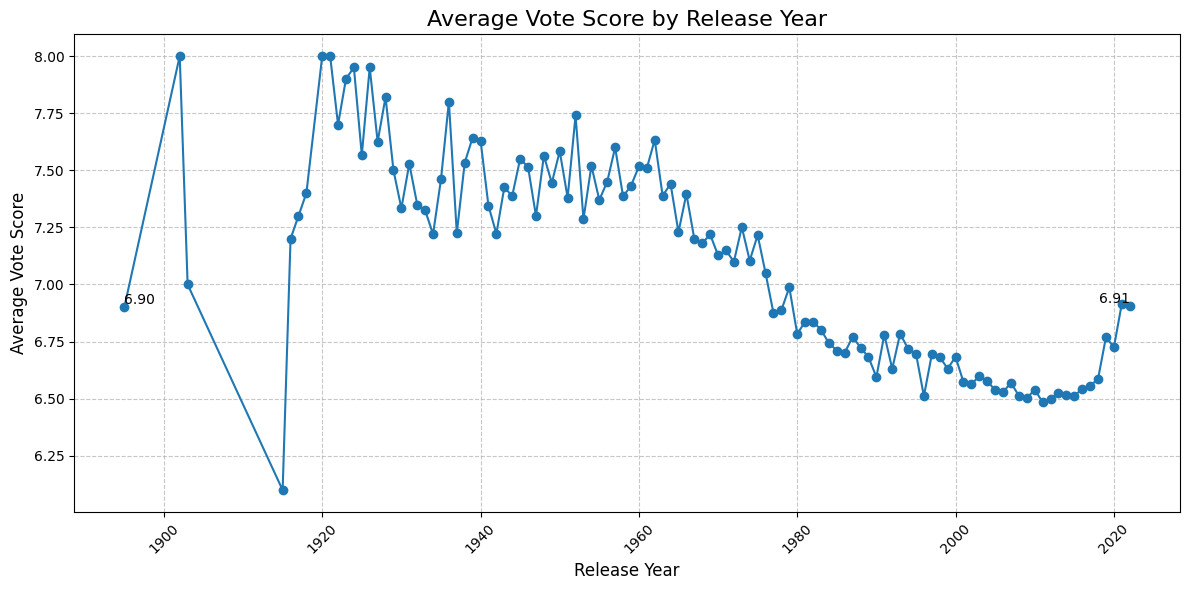
Full final code
Check the full code in JavaScript and Python below:
import 'dotenv/config'
import fs from 'fs'
import Anthropic from '@anthropic-ai/sdk'
import { Sandbox } from '@e2b/code-interpreter'
// Create sandbox
const sbx = await Sandbox.create()
// Upload the dataset to the sandbox
const content = fs.readFileSync('dataset.csv')
const datasetPathInSandbox = await sbx.files.write('/home/user/dataset.csv', content)
async function runAIGeneratedCode(aiGeneratedCode: string) {
const execution = await sbx.runCode(aiGeneratedCode)
if (execution.error) {
console.error('AI-generated code had an error.')
console.log(execution.error.name)
console.log(execution.error.value)
console.log(execution.error.traceback)
process.exit(1)
}
// Iterate over all the results and specifically check for png files that will represent the chart.
let resultIdx = 0
for (const result of execution.results) {
if (result.png) {
// Save the png to a file
// The png is in base64 format.
fs.writeFileSync(`chart-${resultIdx}.png`, result.png, { encoding: 'base64' })
console.log('Chart saved to chart-${resultIdx}.png')
resultIdx++
}
}
}
const prompt = `
I have a CSV file about movies. It has about 10k rows. It's saved in the sandbox at ${datasetPathInSandbox.path}.
These are the columns:
- 'id': number, id of the movie
- 'original_language': string like "eng", "es", "ko", etc
- 'original_title': string that's name of the movie in the original language
- 'overview': string about the movie
- 'popularity': float, from 0 to 9137.939. It's not normalized at all and there are outliers
- 'release_date': date in the format yyyy-mm-dd
- 'title': string that's the name of the movie in english
- 'vote_average': float number between 0 and 10 that's representing viewers voting average
- 'vote_count': int for how many viewers voted
I want to better understand how the vote average has changed over the years. Write Python code that analyzes the dataset based on my request and produces right chart accordingly`
const anthropic = new Anthropic()
console.log('Waiting for the model response...')
const msg = await anthropic.messages.create({
model: 'claude-3-5-sonnet-20240620',
max_tokens: 1024,
messages: [{ role: 'user', content: prompt }],
tools: [
{
name: 'run_python_code',
description: 'Run Python code',
input_schema: {
type: 'object',
properties: {
code: {
type: 'string',
description: 'The Python code to run',
},
},
required: ['code'],
},
},
],
})
interface CodeRunToolInput {
code: string
}
for (const contentBlock of msg.content) {
if (contentBlock.type === 'tool_use') {
if (contentBlock.name === 'run_python_code') {
const code = (contentBlock.input as CodeRunToolInput).code
console.log('Will run following code in the sandbox', code)
// Execute the code in the sandbox
await runAIGeneratedCode(code)
}
}
}